Array Cardio Day 1
이번 강의에서는 할 수 있다고 생각되면 비디오를 중지하고 직접 시도해 보라는 얘기가 나와
할 수 있는 부분들을 해결했다.
아래는 내가 직접 해결해본 코드들이다.
4번은 못 풀었음.
6번은 문제 이해를 못했음.
8번은 해결은 했으나 검색해서 해결.
// Array.prototype.filter()
// 1. Filter the list of inventors for those who were born in the 1500's
const birth = inventors.filter( el => el.year >= 1500 && el.year < 1600 ? el : '' );
console.log(birth);
// Array.prototype.map()
// 2. Give us an array of the inventors first and last names
const names = inventors.map( cur => cur.first + cur.last );
console.log(names);
// Array.prototype.sort()
// 3. Sort the inventors by birthdate, oldest to youngest
const sortToAge = inventors.sort( (a, b) => a.year - b.year );
console.log(sortToAge);
// Array.prototype.reduce()
// 4. How many years did all the inventors live all together?
// 5. Sort the inventors by years lived
const lived = inventors.sort( (a, b) => (b.passed - b.year) - (a.passed - a.year) );
console.log(lived);
// 6. create a list of Boulevards in Paris that contain 'de' anywhere in the name
// https://en.wikipedia.org/wiki/Category:Boulevards_in_Paris
// 7. sort Exercise
// Sort the people alphabetically by last name
const sortToName = people.sort( (a, b) => a.split(/\,\s/)[1].localeCompare(b.split(/\,\s/)[1]) );
console.log(sortToName);
// 8. Reduce Exercise
// Sum up the instances of each of these
const data = ['car', 'car', 'truck', 'truck', 'bike', 'walk', 'car', 'van', 'bike', 'walk', 'car', 'van', 'car', 'truck' ];
const sum = data.reduce(function(acc, cur, idx) {
if(cur in acc) {
acc[cur]++;
} else {
acc[cur] = 1;
}
return acc
}, {});
console.log(sum);
1. 1500년대에 태어난 발명가 목록을 필터링하세요.
const fifteen = inventors.filter(function(inventor) {
if(inventor.year >= 1500 && inventor.year < 1600) {
return true; // keep it
} else {
return false; // true로 반환된 값만 필요하므로 이 else부분은 필요 없음.
}
});
arrow function으로 바꾸기
const fifteen= inventors.filter( inventor => (inventor.year >= 1500 && inventor.year < 1600) );
console.table(fifteen);
console.table은 console창에 table형태로 보여준다.
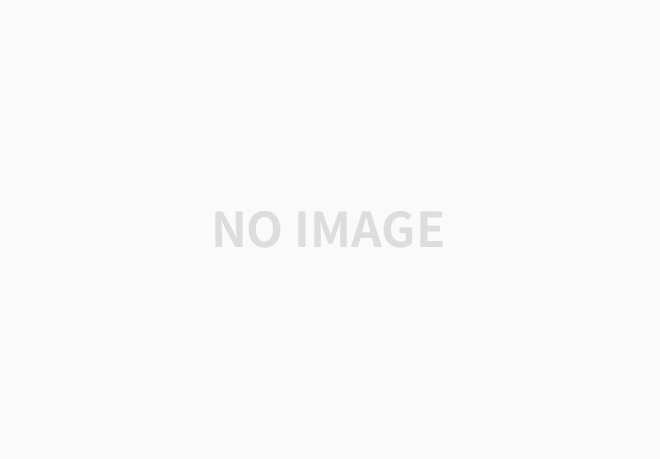
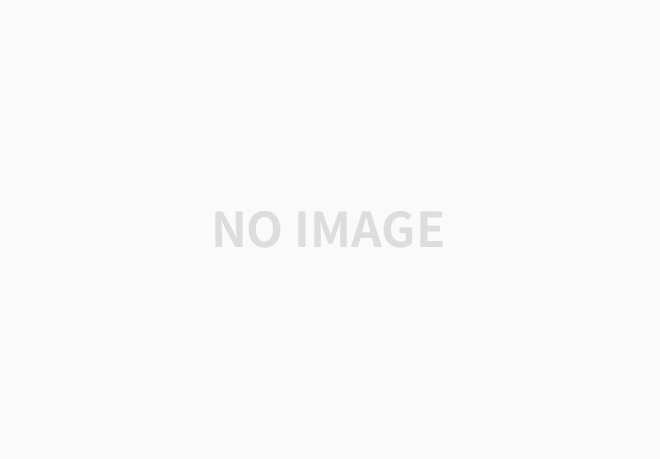
2. 발명가의 이름과 성을 배열로 나타내세요.
//const fullName = inventor.map( inventor => inventor.first + ' ' + inventor.last );
//위 코드를 템플릿 리터럴로 바꿔서 표현
const fullName = inventor.map( inventor => `${inventor.first} ${inventor.last}` );
3. 발명가를 생년월일 기준으로, 가장 나이가 많은 사람부터 가장 나이가 적은 사람 순서로 정렬하세요.
// cont ordred = inventors.sort(function(a, b) {
// if(a.year > b.year) {
// return 1;
// } else {
// return -1;
// }
// });
//위 코드를 arrow function으로 정리
const ordered = inventors.sort( (a, b) => a.year> b.year ? 1 : -1 );
4. 모든 발명가가 살았던 기간은 모두 몇 년입니까?
var totalYears = 0;
for(var i = 0; i < inventors.length; i++) {
totalYears += inventors[i].year
}
const totalYears = inventors.reduce( (total, inventor) => {
return total + (inventor.passed - inventor.year)
}, 0);
arr.reduce(function() {}, 초기값)
초기값은 생략할 수도 있습니다. 하지만 생략하면 원하는 대로 출력되지 않을 수도 있기 때문에 초기값을 입력해 주는 것이 좋습니다.
5. 수명을 기준으로 발명가는 정렬하세요.
// const oldest = inventors.sort(function(a, b) {
// const lastGuy = a.passed - a.year;
// const nextGuy = b.passed - b.year;
// if(lastGuy > nextGuy) {
// return -1;
// } else {
// return 1;
// }
// });
//위 코드를 삼항연산자로 정리
const oldest = inventors.sort(function(a, b) {
const lastGuy = a.passed - a.year;
const nextGuy = b.passed - b.year;
return lastGuy > nextGuy ? -1 : 1;
});
6. 이름에 'de'가 포함된 파리의 거리 목록을 만드세요.
https://en.wikipedia.org/wiki/Category:Boulevards_in_Paris에 들어가면 파리의 거리 이름들이 나옵니다.
이 목록들의 구조를 살펴보면 .mw-category라는 div안에 ul, li로 들어가 있습니다.
const category = document.querySelector('.mw-category');
const links = Array.from(category.querySelectorAll('a')); //카테고리의 모든 a태그를 선택해서 배열로 만들기
const de = links.map(link => link.textContent).filter(streetName => streetName.includes('de'));
// map(link => link.textContent : 배열 links의 각각 요소들에 대해 textContent를 실행해 텍스트를 가져옴
// filter(streetName => streetName.includes('de')) : 가져온 텍스트 중 'de'를 포함하고 있는 요소만 필터
7. people리스트를 성을 기준으로 알파벳 순서로 정렬하세요.
const alpha = people.sort(function (lastOne, nextOne) {
// const parts = lastOne.split(', '); //성과 이름으로 분리하기 위해서 ', '기준으로 문자열을 나눔.
const [aLast, aFirst] = lastOne.split(', '); // const [aLast, aFirst] : 성과 이름의 순서 변경 및 각각을 변수로 지정
const [bLast, bFirst] = nextOne.split(', ');
return aLast > bLast ? 1 : -1;
});
화살표함수로 표현하기
const alpha = people.sort((lastOne, nextOne) => {
const [aLast, aFirst] = lastOne.split(', ');
const [bLast, bFirst] = nextOne.split(', ');
return aLast > bLast ? 1 : -1;
});
8. 각각의 인스턴스를 요약하세요.
const transportation = data.reduce(function(obj, item) {
if(!obj[item]) {
obj[item] = 0;
}
obj[item]++;
return obj;
}, {}) // 초기값이 {}
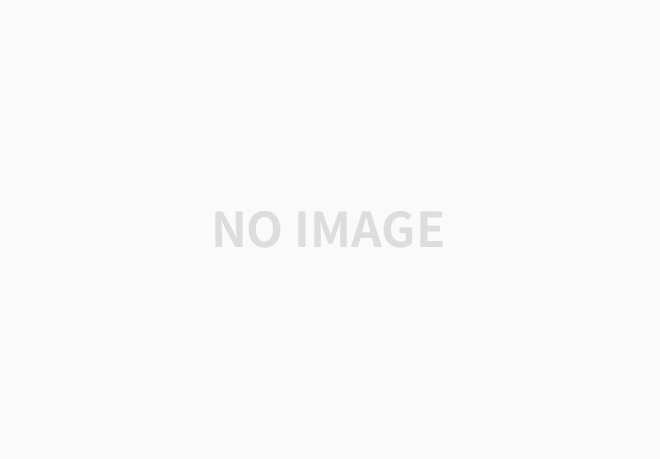
Array.filter() : 조건에 대하여 참인 요소들만 새로운 배열로 반환하는 메서드
Array.map() : 모든 요소에 함수를 실행시켜 얻은 결과값으로 새로운 유형의 배열을 작성하는 메서드
Array.sort() : 요소들을 정렬한 배열을 반환하는 메서드
Array.reduce() : 배열을 하나의 값으로 줄이는 함수를 실행하여 하나의 결괏값을 반환하는 메서드
console.table() : 데이터를 테이블 형식으로 보여주는메서드
JavaScript30 강의를 보고 공부한 글입니다. (https://javascript30.com/)
'JavaScript30 Challenge' 카테고리의 다른 글
[JavaScript30] Day 6 (0) | 2020.04.19 |
---|---|
[JavaScript30] Day 5 (0) | 2020.04.18 |
[JavaScript30] Day 3 (0) | 2020.04.16 |
[JavaScript30] Day 2 (0) | 2020.04.15 |
[JavaScript30] Day 1 (0) | 2020.04.13 |