Array Cardio Day 2
// const isAdult = people.some(function(person) {
// const currentYear = (new Date()).getFullYear();
// if(currentYear - person.year >= 19) {
// return true;
// }
// });
const isAdult = people.some(person => (new Date()).getFullYear() - person.year >= 19);
console.log({isAdult});
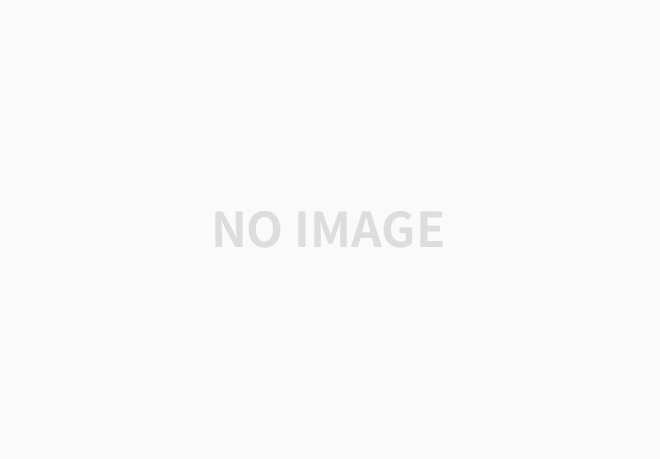
const allAdults = people.every(person => ((new Date()).getFullYear()) - person.year >= 19);
console.log({allAdults});
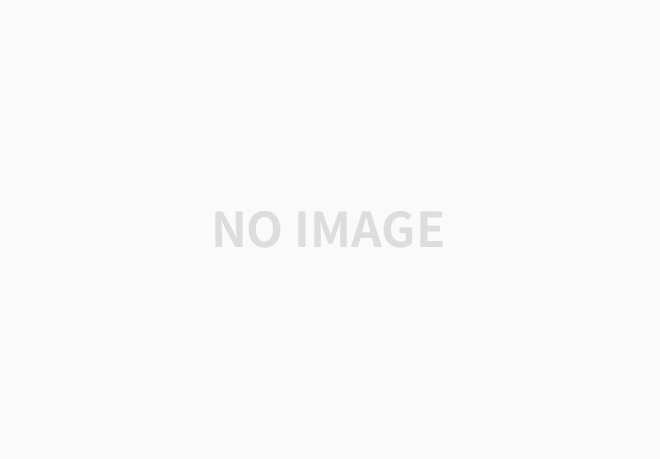
// const comment = commets.find(function(commnet) {
// if(comment.id === 823423) {
// return true;
// }
// });
const comment = comments.find(comment => comment.id === 823423);
console.log(comment)
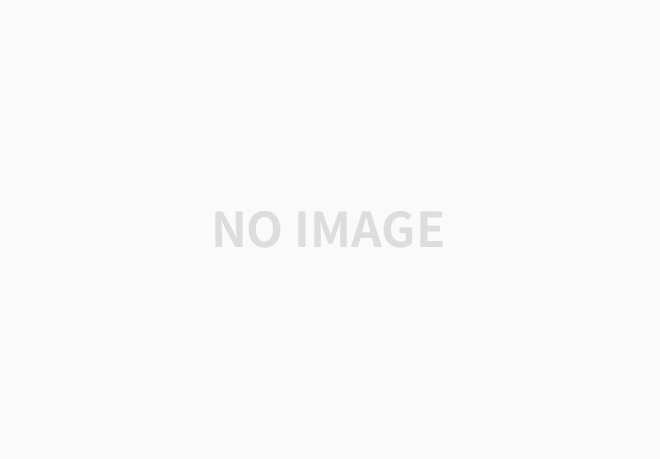
const index = comments.findIndex(comment => comment === 823423);
console.log(index);
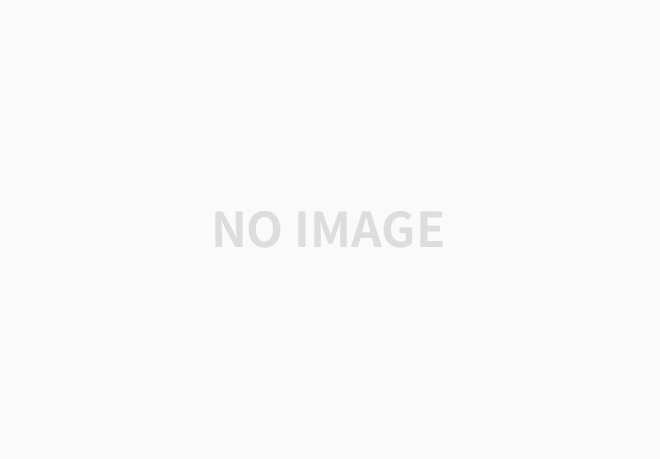
comments.splice(index, 1);
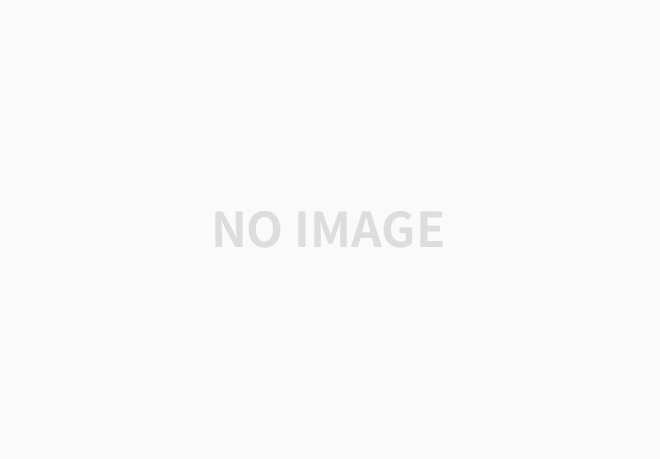
const newComments = [
comments.slice(0, index),
comments.slice(index + 1)
];
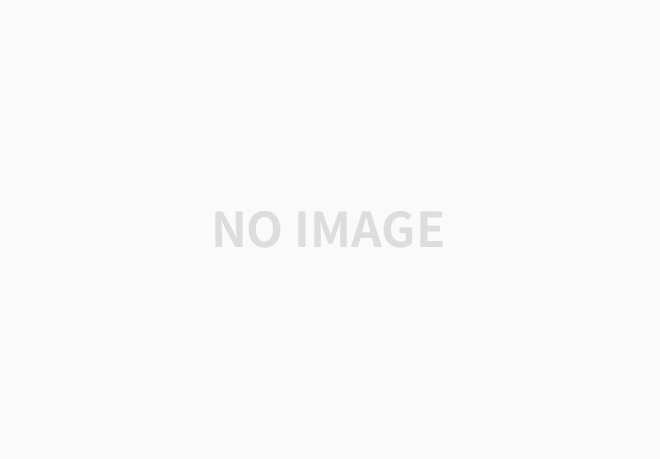
const newComments = [
...comments.slice(0, index),
...comments.slice(index + 1)
];
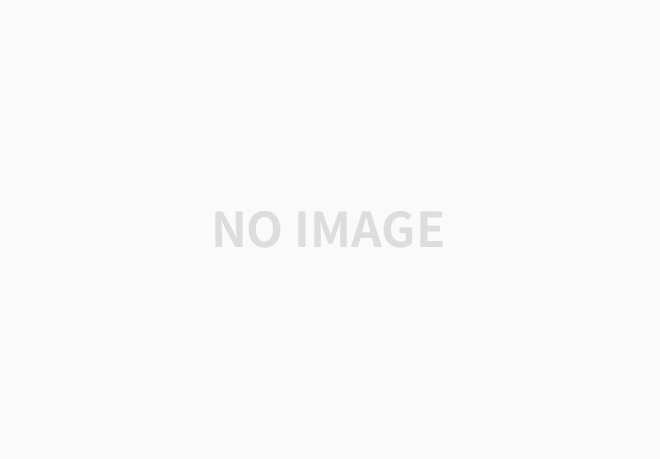
최종 코드
// Some and Every Checks
// Array.prototype.some() // is at least one person 19 or older?
const isAdult = people.some(person => (new Date()).getFullYear() - person.year >= 19);
// Array.prototype.every() // is everyone 19 or older?
const allAdults = people.every(person => ((new Date()).getFullYear()) - person.year >= 19);
// Array.prototype.find()
// Find is like filter, but instead returns just the one you are looking for
// find the comment with the ID of 823423
const comment = comments.find(comment => comment.id === 823423);
// Array.prototype.findIndex()
// Find the comment with this ID
// delete the comment with the ID of 823423
const index = comments.findIndex(comment => comment.id === 823423);
const newComments = [
comments.slice(0, index),
comments.slice(index + 1)
];
Array.some() : 배열 안의 어떤 요소라도 주어진 판별 함수를 통과하는지 테스트하는 메서드
Array.every() : 배열 안의 모든 요소가 주어진 판별 함수를 통과하는지 테스트하는 메서드
Array.find() : 주어진 판별 함수를 만족하는 첫 번째 요소의 값을 반환하는 메서드. 해당 요소 없으면 undefined를 반환.
Array.findIndex() : 주어진 판별 함수를 만족하는 배열의 첫 번 째 요소에 대한 인덱스를 반환. 해당 요소 없으면 -1 반환.
JavaScript30 강의를 보고 공부한 글입니다. (https://javascript30.com/)
'JavaScript30 Challenge' 카테고리의 다른 글
[JavaScript30] Day 9 (0) | 2020.04.26 |
---|---|
[JavaScript30] Day 8 (0) | 2020.04.25 |
[JavaScript30] Day 6 (0) | 2020.04.19 |
[JavaScript30] Day 5 (0) | 2020.04.18 |
[JavaScript30] Day 4 (0) | 2020.04.18 |